일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 정보처리기사
- vue.js 로그인
- vuex
- vue 로그인
- Vue.js 입문
- propsTypes
- 정처기
- React 시작하기
- defaultProps
- router push
- Props
- 함수형 컴포넌트
- 컴포넌트
- 2021 정보처리기사 실기
- Vue.js 시작하기
- 2021 정처기 실기
- State
- 클래스형 컴포넌트
- vuex 새로고침
- router 네비게이션
- 2021 정처기
- router go
- 중첩 라우트
- 라이프사이클
- 2021 정보처리기사
- vue 히스토리 모드
- 백준 1110 시간 초과
- React
- vue.js
- router replace
- Today
- Total
개발 일기
[Vue.js] vuex store 모듈화하기 본문
오늘 글은 아래 글과 이어진다.
2021/01/19 - [Vue.js] - [Vue.js 시작하기] - vuex로 중앙에서 상태 관리 하기
[Vue.js 시작하기] - vuex로 중앙에서 상태 관리 하기
Vuex란? Vue.js 애플리케이션에 사용할 수 있는 상태 관리 라이브러리다. 이를 사용하면 모든 컴포넌트에 대한 중앙 집중식 저장소 역할을 할 수 있다. 상단 인스턴스는 여러개의 많은 하위 컴포넌
developerjournal.tistory.com
모듈화
모듈화를 하는 이유는 클래스도 성격별로 세분화되는 것처럼 이후에 성격이 다른 전역변수가 여러개가 되는 경우를 다루기 위해서다. 또한 부가적인 기능으로는 새로고침을 하면 store의 정보는 사라지게 되는데, 모듈화를 하게 되면 항상 저장하고 싶은 store 변수만 저장할 수 있다. (물론 라이브러리를 사용해야하지만 이는 다음에 다루겠다.)
//src/store/index.js
import Vue from 'vue'
import Vuex from 'vuex'
import axios from "axios";
Vue.use(Vuex)
const HOST = ""
const store = new Vuex.Store({
state: {
animalList: [],
fruitList: []
},
mutations: {
setAnimalList(state, payload) {
state.animalList = payload
},
setFruitList(state, payload) {
state.fruitList = payload
}
},
actions: {
async getAllAnimals({ commit }) {
let response = null
try {
response = await axios(HOST + "/api/getAllAnimal")
}
catch (e) {
console.error(e)
}
commit("setAnimalList", response.data)
},
async getAllFriuts({ commit }) {
let response = null
try {
response = await axios(HOST + "/api/getAllFruits")
}
catch (e) {
console.error(e)
}
commit("setFruitList", response.data)
},
}
})
export default store
위는 이전에 만들어둔 store다. 클래스를 세분화하듯 동물 store와 과일 store를 따로 만들어 보겠다.
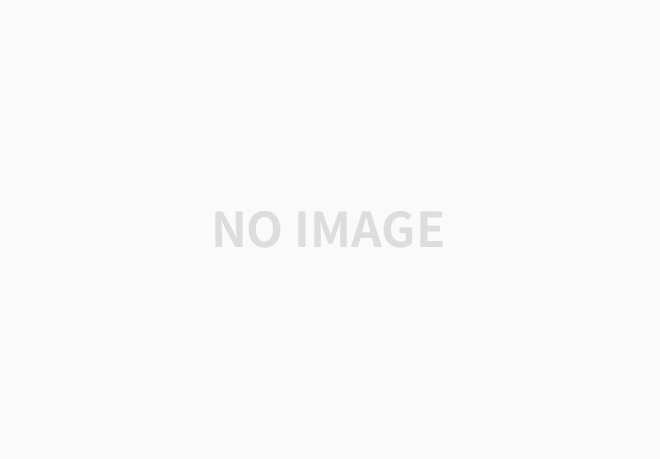
디렉토리 구조는 다음을 참고하면 된다.
animalStore.js
// src/store/modules/animalStore.js
const animalStore = {
state: {
animalList: [],
},
mutations: {
setAnimalList(state, payload) {
state.animalList = payload
},
},
actions: {
async getAllAnimals({ commit }) {
let response = null
try {
response = await axios(HOST + "/api/getAllAnimal")
}
catch (e) {
console.error(e)
}
commit("setAnimalList", response.data)
},
}
}
export default animalStore
animal에 관련된 이전 코드들을 animalStore.js 에 state, mutations, actions 에 맞게 이동하였다.
그리고 export default로 외부에서 사용할 이름을 지정해주면 된다.
fruitStore.js
// src/store/modules/fruitStore.js
const fruitStore = {
state: {
fruitList: []
},
mutations: {
setFruitList(state, payload) {
state.fruitList = payload
}
},
actions: {
async getAllFriuts({ commit }) {
let response = null
try {
response = await axios(HOST + "/api/getAllFruits")
}
catch (e) {
console.error(e)
}
commit("setFruitList", response.data)
},
}
}
export default fruitStore
위와 마찬가지다.
index.js
//src/store/index.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
import animalStore from '@/store/modules/animalStore.js'
import fruitStore from '@/store/modules/fruitStore.js'
const store = new Vuex.Store({
modules: {
//키: 값 형태로 저장
animalStore: animalStore,
fruitStore: fruitStore,
},
})
export default store
위에서 export default 해준 값으로 import를 한 후, store의 modules 옵션에 추가해주면 된다. 이는 키와 값 형태로 저장되므로 앞으로 키를 다른 컴포넌트에서 사용하면 된다.
너무 쉽다..!
다른 컴포넌트에서 vuex 데이터 접근시
다른 store의 구성 요소에 접근하는 방법은 이전과 동일하다. ex) this.$store.dispatch, this.$store.commit 등..
=> 이로 인해서 모듈 내의 action, mutation, getter는 전역 네임스페이스 아래에 등록되었다는 것을 알 수 있다.
=> 만약 모듈이 독립적으로 사용되길 원한다면, namespaced: true라고 각 store에 명시하면 된다.
하지만 state 접근시에는 달라진다.
$store.state.state값 => $store.state.명시된 store 모듈 이름.state값 으로 접근해야한다.
오늘 예제로 보면 $store.state.fruitStore.fruitList 이런식이다.
+ 오늘 알아본 예제뿐아니라 get axios 데이터와 post axios 데이터를 따로 다루는데에도 효율적이다.
'Vue.js' 카테고리의 다른 글
[Vue.js] - 새로고침 후에도 Vuex 상태 유지하기 (vuex-persistedstate 사용) (0) | 2021.04.02 |
---|---|
[Vue.js] 로그인 기능 구현하기 (2) | 2021.03.26 |
[Vue.js] 다국어 처리 (vue-i18n 사용) (0) | 2021.01.21 |
[Vue.js 시작하기] - vuex로 중앙에서 상태 관리 하기 (0) | 2021.01.19 |
[Vue.js 시작하기] - axios 사용하여 서버 통신 하기 (1) | 2021.01.18 |